//字面量
var emptyString = ""
//初始化语法
var anotherEmptyString = ""
//isEmpty 检查是否为空串
if emptyString.isEmpty{print("emptyString isEmpty ")
}
//所谓字面量,就是指像特定的数字,字符串或者是布尔值这样,能够直接了当地指出自己的类型并为变量进行赋值的值。let intNumber = 12 //整型字面量
let hellowString = "Hello!" //字符串字面量
let isBool = true //布尔值字面量//字符串字面量是被双引号包括的固定顺序文本字符、swift会自动推断类型。
let stringContent = "some string"
//多行字符串字面量是用三个双引号引起来的一些列字符,开始和结束默认不会换行符。
let swiftAbout = """
This tutorial guides you through building Landmarks — an app for discovering and sharing the places you love. You’ll start by building the view that shows a landmark’s details.
To lay out the views, Landmarks uses stacks to combine and layer the image and text view components. To add a map to the view, you’ll include a standard MapKit component. As you refine the view’s design, Xcode provides real-time feedback so you can see how those changes translate into code.
Download the project files to begin building this project, and follow the steps below.
"""
print(swiftAbout)
//当你代码中在多行字符串字面量里包含了换行,那么换行符同样会成为字符串里的值。如果你想要使用换行符来让你大妈易读,却不想让换行符成为字符串的值,那就在那些行的末尾使用反斜杠(\)。
let otherSwiftAbout = """
This tutorial guides you through building Landmarks — an app for discovering and sharing the places you love. You’ll start by building the view that shows a landmark’s details.\
To lay out the views, Landmarks uses stacks to combine and layer the image and text view components. To add a map to the view, you’ll include a standard MapKit component. As you refine the view’s design, Xcode provides real-time feedback so you can see how those changes translate into code.\
Download the project files to begin building this project, and follow the steps below.
"""
print(otherSwiftAbout)
//要让多行字符串字面量起始或结束于换行,就在第一个或最后一行写一个空行。
let lineBreaks = """This string starts with a line break.
It also ends with a line break."""
print(lineBreaks)
//多行字符串可以缩进匹配周围的代码。双引号(“”“)前的空格会告诉swift其他行前应该有多少空白是需要忽略的。如果你在某行的空格超过了结束的双引号,那么这些空格会被包含。
let otherLineBreaks = """This string starts with a line break.It also ends with a line break.This string starts with a line break.It also ends with a line break.
"""
print(otherLineBreaks)
//转义字符 \0(空字符)、\\(反斜线)、\t(水平制表符)、\n(换行符)、\r(回车符)、\"(双引号)、\'(单引号)。
//可以在多行字符串字面量中包含双引号(”)而不需要转义。要在多行字符串中包含文本“”“,转义至少一个双引号。
let wiseWords = "\"Imagination is more important than knowledge\" - Einstein"
// "Imageination is more important than knowledge" - Enistein
//Unicode 标量,写成 \u{n}(u 为小写),其中 n 为任意一到八位十六进制数且可用的 Unicode 位码。
let dollarSign = "\u{24}" // $,Unicode 标量 U+0024
let blackHeart = "\u{2665}" // ?,Unicode 标量 U+2665
let sparklingHeart = "\u{1F496}" // ?,Unicode 标量 U+1F496
//在字符串字面量中放置扩展分隔符来在字符串中包含特殊字符而不让它们真的生效。把字符串放在在双引号内并由(#)包括。如果字符有“#则首位需要有两个##。
let rawStr1 = #"Line 1\nLIne 2"#
print(rawStr1)
let rawStr2 = #"Line 1\#nLIne 2"#
print(rawStr2)
//如果你需要字符串中某个特殊符号的效果,使用匹配你包裹的并好数量的并号在前面写转义符号\
//var 指定的可以修改、let指定的不可修改
var variableString = "Horse"
variableString += " and carriage"let otherVariableString = "Horse"
//otherVariableString += " and carriage" //错误:left side of mutating operator isn't mutable
//String 值在传递给方法或者函数的时候会被复制过去,赋值给常量或者变量的时候也是一样。swift编译器优化了字符串使用的资源,实际上拷贝只会在确实需要的时候才会进行。var hlepStr = "help"
var otherHelpStr = hlepStr
print(hlepStr == otherHelpStr)//true
hlepStr += " dog"
print(hlepStr)//help dog
print(otherHelpStr)//help
print(hlepStr == otherHelpStr)//false//操作字符串
//for-in循环便利String中的每一个独立的Character。
for item in "Dog is ?"{print(item)
}
//String值可以通过传入Character数组来构造。
let catCharacter:[Character] = ["C","a","t","!"]
let catString = String(catCharacter)
print(catString)//Cat!
//使用加运算符(+)创建字符串。
var appentStr = "hellow"
appentStr = appentStr + "world"
print(appentStr)//hellowworld
//使用加赋值符号(+=)在已经存在的String值末尾追加一个String值。
appentStr += "!"
print(appentStr)//hellowworld!
//使用String类型的append()方法来可以给一个String变量的末尾追加Character值
appentStr.append("^_^")
print(appentStr)//hellowworld!^_^
//字符串穿插值是一种从混合常量、变量、字面量和表达式的字符串字面量构造新String值的方法。
//每一个你插入字符串字面量的元素都要被一对圆括号包裹,然后使用反斜杠前缀。
let multipier = 2
let message = "\(multipier) time 2.5 is \(Double(multipier) * 2.5)"
//每个string值都有相关的索引类型,String.Index,它相当于每个Character在字符串中的位置。
//starIndex属性访问String中第一个Character的位置。endIndex属性就是String中最后一个字符后的位置。
let greeting = "Guten Tag!"
print(greeting[greeting.startIndex])
//使用index(before:)和index(after:)方法访问给定索引的前后。
print(greeting[greeting.index(before: greeting.endIndex)])//!
print(greeting[greeting.index(after: greeting.startIndex)])//u
//要访问给定索引更远的索引,你可以使用index(:offsetBy:)。使用indices属性访问字符串中每一个字符的索引。
let indexItem = greeting.index(greeting.startIndex,offsetBy:3)
print(greeting[indexItem])
//插入字符串,使用insert(_:at:)方法。
var welcome = "hellow"
welcome.insert("!", at: welcome.endIndex)
//插入另一个字符串的内容到特定的索引,使用inser(contentsOf:at:)方法。
welcome.insert(contentsOf: "there", at: welcome.index(before: welcome.endIndex))
//移字符串,使用remove(at:)方法。
welcome.remove(at: welcome.index(before: welcome.endIndex))
//移除一小段特定范围的字符串,使用removeSubrange(_:)方法。
let rangNewIndex = welcome.index(welcome.endIndex,offsetBy:6)..<welcome.endIndex
welcome.removeSubrange(rangNewIndex)
//使用下标或者类似prefix(_:)的方法得到的子字符串是Substring类型。Substring拥有String的大部分方法。
let winowStr = "Hello,World !"
let winowIndex = winowStr.index(of: ",") ?? winowStr.endIndex
let beginning = winowStr[..<winowIndex]
//Substring可以转换为String
let newWinowStr = String(beginning)
- 子字符串重用一部分原字符串的内存,修改字符串或者子字符串之前都不需要花费拷贝内存的代价。String和Substring都遵循StringProtocol协议,也就是说他基本上能很方便地兼容所有接受StringProtocol值的字符串操作函数。
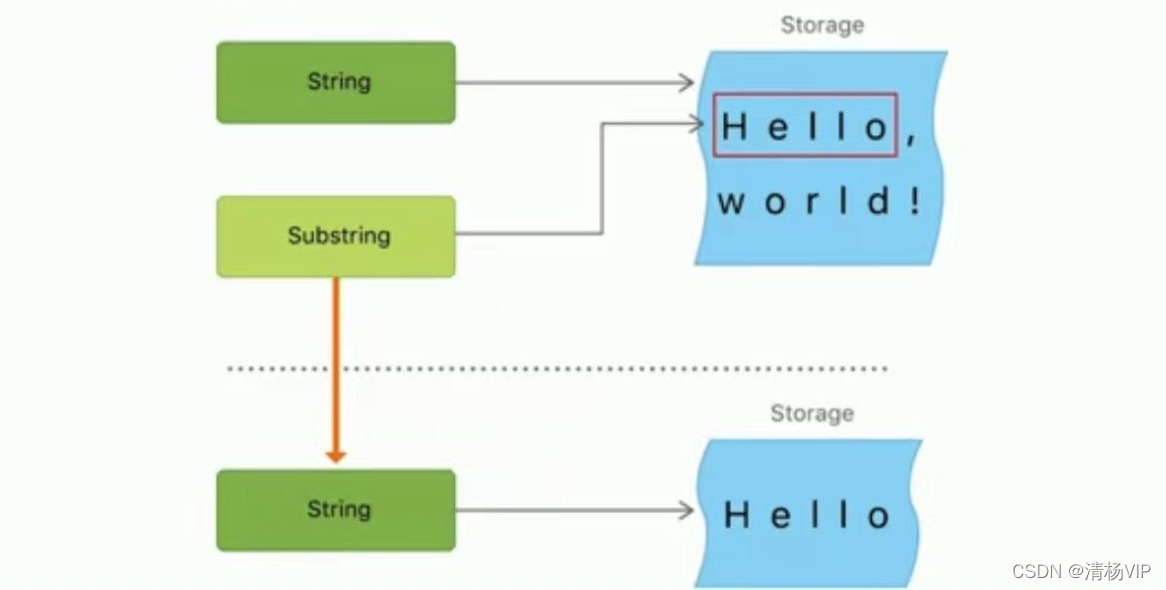
//字符串和字符串相等性(==和!=)
var snowTTSStr = "??"
var snowTTSStr1 = "雪:??"
print(snowTTSStr == snowTTSStr1)//前缀相等hasPreFix(_:),后缀相等性hasSuffix(_:)。
print(snowTTSStr.hasPrefix("??"))
print(snowTTSStr.hasSuffix("??"))