By yangbo 2021/04/12
Pointers 指针
Pointers are also variables(变量) and play a very important role in C programming language. They are used for several(几个) reasons, such as:
-
Strings 字符串
-
Dynamic memory allocation 动态内存分配
-
Sending function arguments by reference 通过引用发送函数参数
-
Building complicated data structures 构建复杂的数据结构
-
Pointing to functions 指向函数
-
Building special data structures (i.e. Tree, Tries, etc...) 构建特殊的数据结构
And many more.
What is a pointer?
A pointer is essentially a simple integer variable which holds a memory address that points to a value, instead of holding the actual value itself. 而不是保存实际值本身
The computer's memory is a sequential(顺序的) store of data, and a pointer points to(指向) a specific part of the memory. Our program can use pointers in such a way that the pointers point to a large amount of memory - depending on how much we decide to read from that point on.
Strings as pointers 字符串作为指针
We've already discussed(讨论) strings, but now we can dive in(深入) a bit deeper and understand what strings in C really are (which are called C-Strings to differentiate them from other strings when mixed with C++)
The following line:
char * name = "John";
does three things:
-
It allocates(分配) a local (stack 堆栈 ) variable(局部变量) called name
, which is a pointer to a single character.
-
It causes the string "John" to appear somewhere in the program memory (after it is compiled and executed, of course).
-
It initializes the name
argument to point to where the J
character resides at (which is followed by the rest of the string in the memory).
If we try to access the name
variable as an array, it will work, and will return the ordinal(序数的) value of the character J
, since the name
variable actually points exactly to the beginning of the string.
Since(因为) we know that the memory is sequential, we can assume that if we move ahead in the memory to the next character(下一个字符), we'll receive the next letter(下一个字母) in the string, until we reach the end of the string, marked with a null terminator(终止符) (the character with the ordinal value of 0, noted as \0
).
Dereferencing 取消引用
Dereferencing is the act of referring to where the pointer points, instead of the memory address(内存地址). We are already using dereferencing in arrays - but we just didn't know it yet. The brackets operator - [0]
for example, accesses the first item of the array. And since arrays are actually pointers, accessing the first item in the array is the same as dereferencing a pointer. Dereferencing a pointer is done using the asterisk operator *
.
If we want to create an array that will point to a different variable in our stack, we can write the following code:
/* define a local variable a */
int a = 1;/* define a pointer variable, and point it to a using the & operator */
int * pointer_to_a = &a;printf("The value a is %d\n", a);
printf("The value of a is also %d\n", *pointer_to_a);
Notice that we used the &
operator to point at the variable a
, which we have just created.
We then referred to(引用) it using the dereferencing operator. We can also change the contents of the dereferenced variable:
int a = 1;
int * pointer_to_a = &a;/* let's change the variable a */
a += 1;/* we just changed the variable again! */
*pointer_to_a += 1;/* will print out 3 */
printf("The value of a is now %d\n", a);
Exercise
Create a pointer to the local variable n
called pointer_to_n
, and use it to increase the value of n
by one.
原:
#include <stdio.h>int main() {int n = 10;/* your code goes here *//* testing code */if (pointer_to_n != &n) return 1;if (*pointer_to_n != 11) return 1;printf("Done!\n");return 0;
}
改:
#include <stdio.h>int main() {int n = 10;int * pointer_to_n = &n;*pointer_to_n += 1;/* testing code */if (pointer_to_n != &n) return 1;if (*pointer_to_n != 11) return 1;printf("Done!\n");return 0;
}
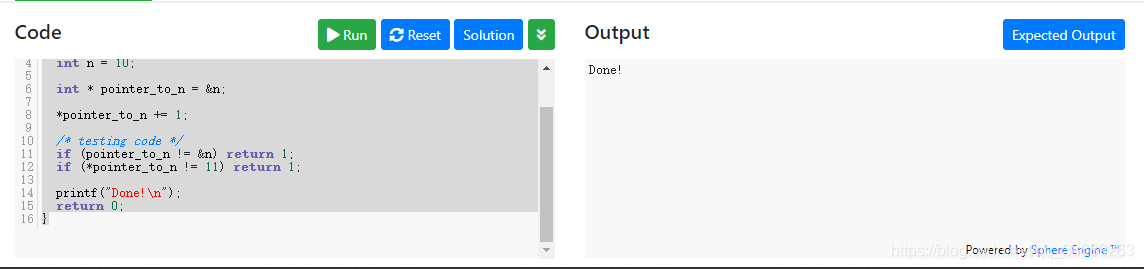