在JavaScript中有好几种方法都可以实现继承。原型继承——使用prototype作为一种继承机制有许多优点,下面举例:
function Parent() { var parentPrivate = "parent private data"; var that = this; this.parentMethodForPrivate = function () { return parentPrivate; }; console.log("parent"); } Parent.prototype = { parentData: "parent data", parentMethod: function (arg) { return "parent method"; }, overrideMethod: function (arg) { return arg + " overriden parent method"; } } function Child() { // super constructor is not called, we have to invoke it Parent.call(this); console.log(this.parentData); var that = this; this.parentPrivate = function () { return that.parentMethodForPrivate(); }; console.log("child"); } //inheritance Child.prototype = new Parent(); // parent Child.prototype.constructor = Child; //lets add extented functions Child.prototype.extensionMethod = function () { return " child’ s" + this.parentData; }; //override inherited functions Child.prototype.overrideMethod = function () { //parent’s method is called return" Invoking from child " + Parent.prototype.overrideMethod.call(this, "test"); }; var child = new Child(); // parent // parent data // child console.log(child.extensionMethod()); //child’s parent data console.log(child.parentData); //parent data console.log(child.parentMethod()); //parent method console.log(child.overrideMethod()); //Invoking from child test overriden parent method console.log(child.parentPrivate()); // parent private data console.log(child instanceof Parent); //true console.log(child instanceof Child); //true
当访问子对象的成员时,从子对象开始找起,如果找不到则在其原型对象中搜索。如果还找不到,则在其父类对象和原型中搜索。以此类推一直搜索到Object的原型。这种层次被称之为"原型链"。下图描述了这种关系:
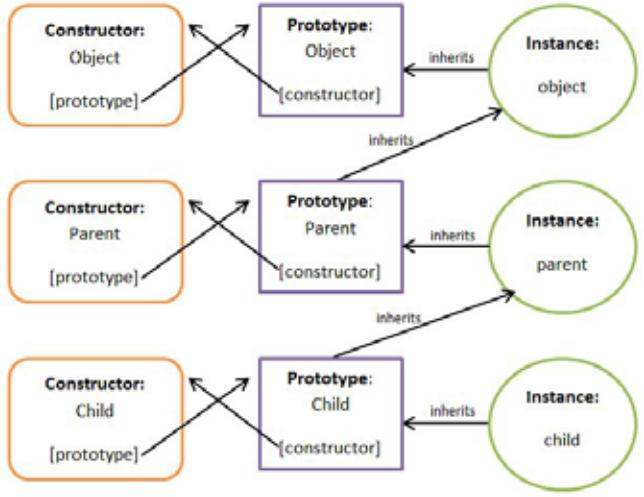