自己写的五子棋,之前运行都还可以,最近更新了个显卡驱动,再重新打开就出现问题
代码如下:
package game;
//五子棋
import java.awt.BorderLayout;
import java.awt.Button;
import java.awt.FlowLayout;
import java.awt.Graphics;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JPanel;
public class MyFive{
private JFrame frame;
private Chessboard cb;
private Graphics g;
private JDialog d;
private JLabel jl;
private Button jb;
private JMenuBar menuBar;
private JMenu game,help;
private JMenuItem newGame,pause,goOn,exit,about;
private String winner;
private MyMouseAdapter mma;
int[][] chess=new int[11][11];
private int time=0; //回合数
public void init(){
frame=new JFrame("五子棋");
frame.setBounds(50,50,300,300);
frame.setLayout(new BorderLayout());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
d=new JDialog(frame,"游戏结束");
d.setSize(200, 100);
d.setLayout(new FlowLayout());
jl=new JLabel();
jb=new Button("退出");
d.add(jl);
d.add(jb);
cb=new Chessboard();
g=cb.getGraphics();
frame.add(cb,BorderLayout.CENTER);
menuBar=new JMenuBar();
game=new JMenu("游戏");
newGame=game.add("新游戏");
pause=game.add("暂停");
goOn=game.add("继续");
exit=game.add("退出");
help=new JMenu("帮助");
about=help.add("关于");
menuBar.add(game);
menuBar.add(help);
frame.setJMenuBar(menuBar);
// frame.add(menuBar);
mma=new MyMouseAdapter();
}
public MyFive(){
init();
myEvent();
}
class MyMouseAdapter extends MouseAdapter{
public void mouseClicked(MouseEvent e){
int x=e.getX();
int y=e.getY();
int hang,lie;
hang=(int) Math.round(y/20.0); //行
lie=(int)Math.round(x/20.0); //列
for(int i=1;i<11;i++){
for(int j=1;j<11;j++){
if(chess[i][j]!=0)
time++; //通过计算矩阵里面有几个非零数来确定下了多少次
}
}
if(time%2==0&&x>10&&x<210&&y>10&&y<210){ //偶数 黑棋下
if(chess[hang][lie]==0) //没下过的地方
{
chess[hang][lie]=1; //将黑棋下的地方改为1
// System.out.println(time+"---------------");
// show();
}
}
if(time%2==1&&x>10&&x<210&&y>10&&y<210)//基数 白棋下
{
if(chess[hang][lie]==0) //没下过的地方
{
chess[hang][lie]=2; //将黑棋下的地方改为2
// System.out.println(time+"---------------");
// show();
}
}
cb.repaint();
// System.out.println("hang:"+hang);
// System.out.println("lie:"+lie);
if(isWin(hang,lie)){
if(chess[hang][lie]==1){
winner="黑方";
}else{
winner="白方";
}
jl.setText(winner+"胜");
d.setVisible(true);
// System.out.println("game over");
}
time=0; //time归零,避免下一次计数时用上次的数据
}
}
public void myEvent(){
cb.addMouseListener(mma);
jb.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent arg0) {
System.exit(0);
}
});
newGame.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent arg0) {
for(int i=0;i<11;i++)
for(int j=0;j<11;j++)
chess[i][j]=0;
cb.repaint();
time=0; //time要改为原先的设置,否则第二次运行时会出错
}
});
pause.addActionListener(new ActionListener(){ //暂停就移除监听器
public void actionPerformed(ActionEvent arg0) {
cb.removeMouseListener(mma);
}
});
goOn.addActionListener(new ActionListener(){ //继续就添加监听器
public void actionPerformed(ActionEvent arg0) {
cb.addMouseListener(mma);
}
});
exit.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent arg0) {
System.exit(0);
}
});
}
public boolean isWin(int hang,int lie){ //判断游戏是否结束
int i_temp=chess[hang][lie];
int count=1;
int x=hang;
int y=lie;
//横向判断
while(x>0 && i_temp==chess[--x][y]) count++;
x=hang; //每次运行完都要将x重新复位,否则会带着上次运算的值进行
while(x<9 && i_temp==chess[++x][y]) count++;
if(count>=5)
return true;
//纵向判断
count=1;
x=hang;
y=lie;
while(y>0 && i_temp==chess[x][--y]) count++;
y=lie;
while(y<9 && i_temp==chess[x][++y]) count++;
if(count>=5)
return true;
//斜向判断1
count=1;
x=hang;
y=lie;
while(y>0 && x>0 && i_temp==chess[--x][--y]) count++;
x=hang;
y=lie;
while(y<9 && x<9 && i_temp==chess[++x][++y]) count++;
if(count>=5)
return true;
//斜向判断2
count=1;
x=hang;
y=lie;
while(y>0 && x<9 && i_temp==chess[++x][--y]) count++;
x=hang;
y=lie;
while(y<9 && x>0 && i_temp==chess[--x][++y]) count++;
if(count>=5)
return true;
return false;
}
public void show(){ //打印棋盘矩阵
for(int i=1;i<11;i++){
for(int j=1;j<11;j++){
System.out.print(chess[i][j]+" ");
}
System.out.println();
}
}
class Chessboard extends JPanel//棋盘面板
{
public void paintComponent(Graphics g){
for(int i=1;i<10;i++){
for(int j=1;j<10;j++){
g.drawRect(i*20, j*20, 20, 20);
}
}
for(int i=1;i<11;i++){
for(int j=1;j<11;j++){
if(chess[i][j]==1){ //黑棋
g.fillOval(j*20-7, i*20-7, 15, 15); //对圆心进行微调
}
if(chess[i][j]==2){ //白棋
g.drawOval(j*20-7, i*20-7, 15, 15);
}
}
}
}
}
public static void main(String[] args) {
MyFive five=new MyFive();
}
}
一开始打开
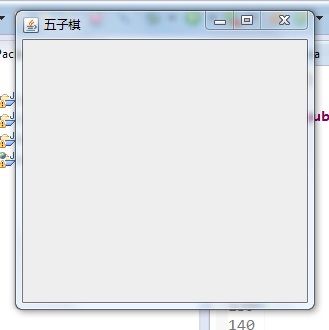
有时候打开时这样的
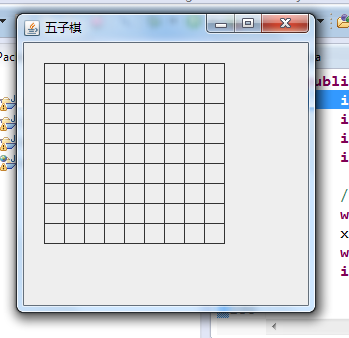
把边框拉大一下,
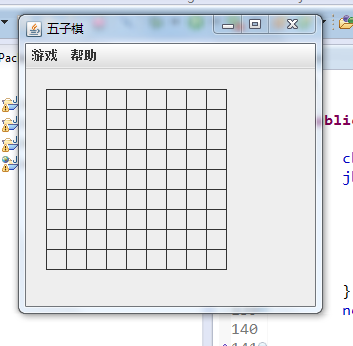
点击就出问题了
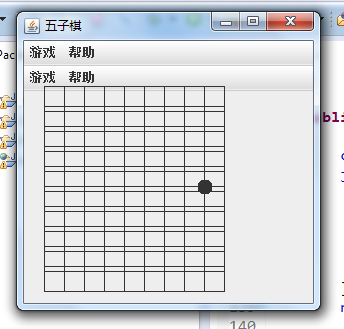
有时候点多了还会报错
Exception in thread "AWT-EventQueue-0" java.lang.ArrayIndexOutOfBoundsException: 12
at game.MyFive.isWin(MyFive.java:175)
at game.MyFive$MyMouseAdapter.mouseClicked(MyFive.java:114)
at java.awt.Component.processMouseEvent(Unknown Source)
at javax.swing.JComponent.processMouseEvent(Unknown Source)
at java.awt.Component.processEvent(Unknown Source)
at java.awt.Container.processEvent(Unknown Source)
at java.awt.Component.dispatchEventImpl(Unknown Source)
at java.awt.Container.dispatchEventImpl(Unknown Source)
at java.awt.Component.dispatchEvent(Unknown Source)
at java.awt.LightweightDispatcher.retargetMouseEvent(Unknown Source)
at java.awt.LightweightDispatcher.processMouseEvent(Unknown Source)
at java.awt.LightweightDispatcher.dispatchEvent(Unknown Source)
at java.awt.Container.dispatchEventImpl(Unknown Source)
at java.awt.Window.dispatchEventImpl(Unknown Source)
at java.awt.Component.dispatchEvent(Unknown Source)
at java.awt.EventQueue.dispatchEventImpl(Unknown Source)
at java.awt.EventQueue.access$400(Unknown Source)
at java.awt.EventQueue$3.run(Unknown Source)
at java.awt.EventQueue$3.run(Unknown Source)
at java.security.AccessController.doPrivileged(Native Method)
at java.security.ProtectionDomain$1.doIntersectionPrivilege(Unknown Source)
at java.security.ProtectionDomain$1.doIntersectionPrivilege(Unknown Source)
at java.awt.EventQueue$4.run(Unknown Source)
at java.awt.EventQueue$4.run(Unknown Source)
at java.security.AccessController.doPrivileged(Native Method)
at java.security.ProtectionDomain$1.doIntersectionPrivilege(Unknown Source)
at java.awt.EventQueue.dispatchEvent(Unknown Source)
at java.awt.EventDispatchThread.pumpOneEventForFilters(Unknown Source)
at java.awt.EventDispatchThread.pumpEventsForFilter(Unknown Source)
at java.awt.EventDispatchThread.pumpEventsForHierarchy(Unknown Source)
at java.awt.EventDispatchThread.pumpEvents(Unknown Source)
at java.awt.EventDispatchThread.pumpEvents(Unknown Source)
at java.awt.EventDispatchThread.run(Unknown Source)
没更新驱动之前就是拉一下边框就出来,其他的都正常,功能都能实现,是什么原因?
求高手指点那些地方出错,应该怎样改,如何完善。如果有更好的代码也可以发到我的邮箱934427310@qq.com。万分感激。
------解决思路----------------------
public void paintComponent(Graphics g)里调用下
super.paintComponent(g);
每次重绘清下屏就可以了
------解决思路----------------------
每次重绘清下屏就可以了
------解决思路----------------------
Swing在java的GUI编程里面已经逐步淡出了,建议尽快转到Jface/AWT编程上面来
------解决思路----------------------
初始化里面你是先显示后加菜单项,当然得手动拉一下,重绘一次才能显示菜单
要想一运行就显示菜单
把frame.setVisible(true);移到最后去