这是还书窗体
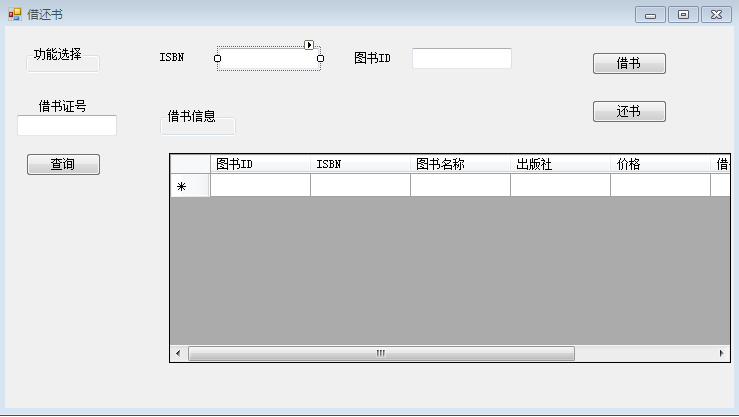
这是还书按钮下的代码(包括用到的函数)
private void button2_Click(object sender, EventArgs e)
{
if (textBox1.Text.Trim() == "" || textBox2.Text.Trim() == "" || textBox3.Text.Trim() == "")
{
MessageBox.Show("借书证号,ISBN,图书ID输入完整!");
return;
}
SqlConnection conn = new SqlConnection(strcon);
SqlCommand cmd = new SqlCommand("Book_Borrow", conn);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter inReaderID = new SqlParameter("@in_ReaderID", SqlDbType.Char, 8);
inReaderID.Direction = ParameterDirection.Input;
inReaderID.Value = textBox1.Text.Trim();
cmd.Parameters.Add(inReaderID);
SqlParameter inISBN = new SqlParameter("@in_ISBN", SqlDbType.Char, 18);
inISBN.Direction = ParameterDirection.Input;
inISBN.Value = textBox2.Text.Trim();
cmd.Parameters.Add(inISBN);
SqlParameter inBookID = new SqlParameter("@in_BookID", SqlDbType.Char, 8);
inBookID.Direction = ParameterDirection.Input;
inBookID.Value = textBox3.Text.Trim();
cmd.Parameters.Add(inBookID);
//SqlParameter inLTime = new SqlParameter("@in_LTime", SqlDbType.DateTime);
//inLTime.Direction = ParameterDirection.Input;
//inLTime.Value = textBox4.Text.Trim();
//cmd.Parameters.Add(inLTime);
SqlParameter outReturn = new SqlParameter("@out_str", SqlDbType.Char, 30);
outReturn.Direction = ParameterDirection.Output;
cmd.Parameters.Add(outReturn);
try
{
conn.Open();
cmd.ExecuteNonQuery();
MessageBox.Show(outReturn.Value.ToString());
}
catch
{ MessageBox.Show("借书出错!"); }
finally
{
conn.Close();
button1_Click(null, null);
}
}
private void button3_Click(object sender, EventArgs e)
{
DataRow[] rows = this.GetSpecialRecord(this.textBox1.Text, this.textBox2.Text, this.textBox3.Text);
if (rows != null)
{
foreach (DataRow drow in rows)
{
int dday = System.DateTime.Today.DayOfYear - ((System.DateTime)drow["RTime"]).DayOfYear;
if (dday > 0)
if (MessageBox.Show(this.textBox1.Text + "客户你的" + this.textBox2.Text + "," + this.textBox3.Text + "图书已经过期" + Convert.ToString(dday)
+ "天,罚 款" + Convert.ToString(dday / 10) + "元RMB", "过期", MessageBoxButtons.OKCancel, MessageBoxIcon.Warning) == DialogResult.Cancel)
{
return;
}
drow.Delete();
}
}
if (ReturnReader(this.textBox1.Text) && ReturnBook(this.textBox2.Text, this.textBox2.Text))
{
UpdateDataSet();
dataGridView1.Refresh();
MessageBox.Show(this.textBox1.Text + "归还图书" + this.textBox2.Text + "成功");
}
}
public void UpdateDataSet()
{
LibraryDataSet5 objDataSetTemp = new LibraryDataSet5();
objDataSetTemp = (LibraryDataSet5)(this.libraryDataSet5.GetChanges());
try
{
this.UpdateDataSource(objDataSetTemp);
this.libraryDataSet5.Merge(objDataSetTemp);
this.libraryDataSet5.AcceptChanges();
}
catch (System.Exception E)
{
this.ErrorHandle(E);
}
}
public void UpdateDataSource(LibraryDataSet5 Changerows)
{
SqlConnection conn = new SqlConnection(strcon);
string sqlStrSelect = "delete [BookID],[ISBN],[BookName],[Publisher],[Price],[Ltime] from [RBL] where [ReaderID]='" + textBox1.Text.Trim() + "'";
try
{
SqlDataAdapter adapter = new SqlDataAdapter(sqlStrSelect, conn);
conn.Open();
adapter.Update(Changerows);
}
catch (System.Exception E)
{
this.ErrorHandle(E);
}
finally
{
conn.Close();
}
}
public DataRow[] GetSpecialRecord(string ReaderID, string ISBN, string BookID)
{
try
{
DataRow[] rows = this.libraryDataSet5.Tables["RBL"].Select("ReaderID= '" + ReaderID + "'" + "and ISBN ='" + ISBN + "'" + "and BookID='" + BookID + "'");
return rows;
}
catch (Exception ex)
{
Console.WriteLine("GetSpecialExpert failed in ExpertAccess!" + ex.StackTrace.ToString());
return null;
}
}
private bool ReturnReader(string ReaderID)
{
SqlConnection conn = new SqlConnection(strcon);
SqlCommand returnbook = new SqlCommand();
returnbook.Connection = conn;
returnbook.CommandType = CommandType.StoredProcedure;
returnbook.CommandText = "Return_Reader";
SqlParameter parinput = returnbook.Parameters.Add("@ReaderID", SqlDbType.Char);
parinput.Direction = ParameterDirection.Input;
parinput.Value = ReaderID;
try
{
conn.Open();
returnbook.ExecuteNonQuery();
conn.Close();
return true;
}
catch (System.Exception e)
{
this.ErrorHandle(e);
conn.Close();
return false;
}
}
//private void dataGridView1_CurrentCellChanged(object sender, System.EventArgs e)
//{
// this.textBox1.Text = this.dataGridView1.CurrentCell.ToString();
// this.textBox1.Refresh();
//}
private bool ReturnBook(string ISBN, string BookID)
{
SqlConnection conn = new SqlConnection(strcon);
SqlCommand returnbook = new SqlCommand();
returnbook.Connection = conn;
returnbook.CommandType = CommandType.StoredProcedure;
returnbook.CommandText = "Return_Book";
SqlParameter parinput = returnbook.Parameters.Add("@ISBN", SqlDbType.Char);
parinput.Direction = ParameterDirection.Input;
parinput.Value = ISBN;
SqlParameter input = returnbook.Parameters.Add("@BookID", SqlDbType.Char);
input.Direction = ParameterDirection.Input;
input.Value = BookID;
try
{
conn.Open();
returnbook.ExecuteNonQuery();
conn.Close();
return true;
}
catch (System.Exception e)
{
this.ErrorHandle(e);
conn.Close();
return false;
}
}
private void ErrorHandle(System.Exception E)
{
MessageBox.Show(E.ToString());
}
执行时出现这种问题
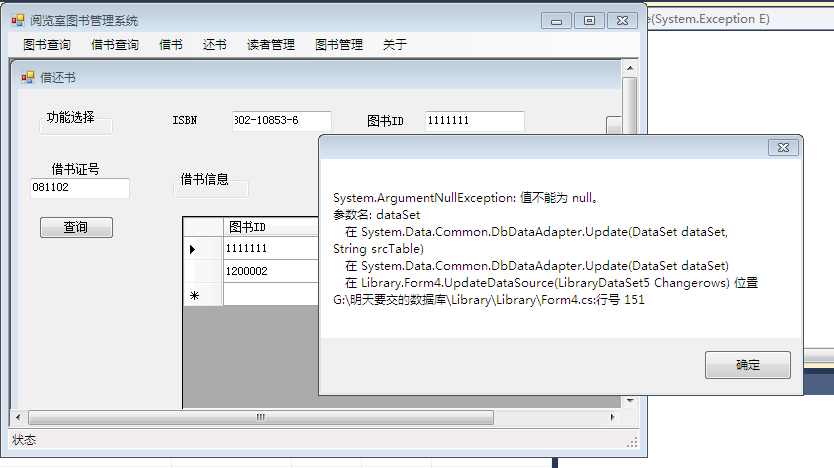
该如何解决呢?????
------解决思路----------------------
只能肯定this.libraryDataSet5.GetChanges()这个返回了null
------解决思路----------------------
调试啊