本讲内容:Service
1、Service的概念
2、Service的生命周期
3、实例:控制音乐播放的Service
本讲源代码:Android学习指南第十四讲源代码
一、Service的概念
Service是Android程序中四大基础组件之一,它和Activity一样都是Context的子类,只不过它没有UI界面,是在后台运行的组件。
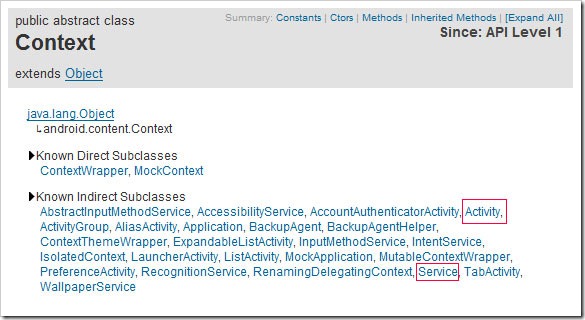
二、Service的生命周期
Service对象不能自己启动,需要通过某个Activity、Service或者其他Context对象来启动。启动的方法有两种,Context.startService和Context.bindService()。两种方式的生命周期是不同的,具体如下所示。
Context.startService方式的生命周期:
启动时,startService –> onCreate() –> onStart()
停止时,stopService –> onDestroy()
Context.bindService方式的生命周期:
绑定时,bindService? -> onCreate() –> onBind()
解绑定时,unbindService –>onUnbind() –> onDestory()
三、实例:控制音乐播放的Service
下面我们用一个可以控制在后台播放音乐的例子来演示刚才所学知识,同学们可以通过该例子可以明显看到通过绑定方式运行的Service在绑定对象被销毁后也被销毁了。
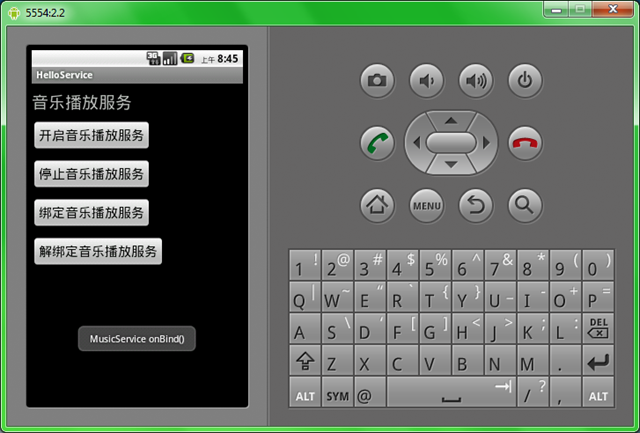
下面把代码分享如下:
1、建立一个新项目名字叫 Lesson14_HelloService,Activity起名叫MainHelloService.java
2、res/layout/main.xml中代码写成
01 | < ? xml version = "1.0" encoding = "utf-8" ?> |
02 | < LINEARLAYOUT android:layout_height = "fill_parent" android:layout_width = "fill_parent" android:orientation = "vertical" xmlns:android = "http://schemas.android.com/apk/res/android" > |
03 | < TEXTVIEW android:layout_height = "wrap_content" android:layout_width = "fill_parent" android:layout_marginTop = "10dp" android:textSize = "25sp" android:text = "音乐播放服务" /> |
04 | < BUTTON type = submit android:layout_height = "wrap_content" android:layout_width = "wrap_content" android:layout_marginTop = "10dp" android:textSize = "20sp" android:text = "开启音乐播放服务" android:id = "@+id/Button01" > |
06 | < BUTTON type = submit android:layout_height = "wrap_content" android:layout_width = "wrap_content" android:layout_marginTop = "10dp" android:textSize = "20sp" android:text = "停止音乐播放服务" android:id = "@+id/Button02" > |
09 | < BUTTON type = submit android:layout_height = "wrap_content" android:layout_width = "wrap_content" android:layout_marginTop = "10dp" android:textSize = "20sp" android:text = "绑定音乐播放服务" android:id = "@+id/Button03" > |
11 | < BUTTON type = submit android:layout_height = "wrap_content" android:layout_width = "wrap_content" android:layout_marginTop = "10dp" android:textSize = "20sp" android:text = "解绑定音乐播放服务" android:id = "@+id/Button04" > |
2、在res目录中建立一个raw目录,并把一个音乐文件babayetu.mp3拷贝进来
3、在Activity的同目录新建一个service文件MusicService.java
01 | package android.basic.lesson14; |
03 | import android.app.Service; |
04 | import android.content.Intent; |
05 | import android.media.MediaPlayer; |
06 | import android.os.IBinder; |
07 | import android.util.Log; |
08 | import android.widget.Toast; |
10 | public class MusicService extends Service { |
13 | ???? String tag = "MusicService" ;? |
16 | ???? MediaPlayer mPlayer; |
20 | ???? public IBinder onBind(Intent intent) { |
21 | ???????? Toast.makeText( this , "MusicService onBind()" ,Toast.LENGTH_SHORT).show(); |
22 | ???????? Log.i(tag, "MusicService onBind()" ); |
23 | ???????? mPlayer.start(); |
29 | ???? public boolean onUnbind(Intent intent){ |
30 | ???????? Toast.makeText( this , "MusicService onUnbind()" , Toast.LENGTH_SHORT).show(); |
31 | ???????? Log.i(tag, "MusicService onUnbind()" ); |
32 | ???????? mPlayer.stop(); |
38 | ???? public void onCreate(){ |
39 | ???????? Toast.makeText( this , "MusicService onCreate()" , Toast.LENGTH_SHORT).show(); |
41 | ???????? mPlayer=MediaPlayer.create(getApplicationContext(), R.raw.babayetu); |
43 | ???????? mPlayer.setLooping( true ); |
44 | ???????? Log.i(tag, "MusicService onCreate()" ); |
49 | ???? public void onStart(Intent intent, int startid){ |
50 | ???????? Toast.makeText( this , "MusicService onStart" ,Toast.LENGTH_SHORT).show(); |
51 | ???????? Log.i(tag, "MusicService onStart()" ); |
52 | ???????? mPlayer.start(); |
57 | ???? public void onDestroy(){ |
58 | ???????? Toast.makeText( this , "MusicService onDestroy()" , Toast.LENGTH_SHORT).show(); |
59 | ???????? mPlayer.stop(); |
60 | ???????? Log.i(tag, "MusicService onDestroy()" ); |
4、MainHelloService.java中的代码:
?
01 | package android.basic.lesson14; |
03 | import android.app.Activity; |
04 | import android.content.ComponentName; |
05 | import android.content.Context; |
06 | import android.content.Intent; |
07 | import android.content.ServiceConnection; |
08 | import android.os.Bundle; |
09 | import android.os.IBinder; |
10 | import android.util.Log; |
11 | import android.view.View; |
12 | import android.view.View.OnClickListener; |
13 | import android.widget.Button; |
14 | import android.widget.Toast; |
16 | public class MainHelloService extends Activity { |
19 | ???? String tag = "MusicService" ; |
21 | ???? /** Called when the activity is first created. */ |
23 | ???? public void onCreate(Bundle savedInstanceState) { |
24 | ???????? super .onCreate(savedInstanceState); |
25 | ???????? setContentView(R.layout.main); |
28 | ???????? Toast.makeText(MainHelloService. this , "MainHelloService onCreate" , Toast.LENGTH_SHORT).show(); |
29 | ???????? Log.i(tag, "MainHelloService onCreate" ); |
32 | ???????? Button b1= (Button)findViewById(R.id.Button01); |
33 | ???????? Button b2= (Button)findViewById(R.id.Button02); |
34 | ???????? Button b3= (Button)findViewById(R.id.Button03); |
35 | ???????? Button b4= (Button)findViewById(R.id.Button04); |
38 | ????????? final ServiceConnection conn = new ServiceConnection(){ |
41 | ???????????? public void onServiceConnected(ComponentName name, IBinder service) { |
42 | ???????????????? Toast.makeText(MainHelloService. this , "ServiceConnection onServiceConnected" , Toast.LENGTH_SHORT).show(); |
43 | ???????????????? Log.i(tag, "ServiceConnection onServiceConnected" ); |
48 | ???????????? public void onServiceDisconnected(ComponentName name) { |
49 | ???????????????? Toast.makeText(MainHelloService. this , "ServiceConnection onServiceDisconnected" , Toast.LENGTH_SHORT).show(); |
50 | ???????????????? Log.i(tag, "ServiceConnection onServiceDisconnected" ); |
55 | ???????? OnClickListener ocl= new OnClickListener(){ |
58 | ???????????? public void onClick(View v) { |
60 | ???????????????? Intent intent = new Intent(MainHelloService. this ,android.basic.lesson14.MusicService. class ); |
61 | ???????????????? switch (v.getId()){ |
62 | ???????????????? case R.id.Button01: |
64 | ???????????????????? startService(intent); |
65 | ???????????????????? break ; |
66 | ???????????????? case R.id.Button02: |
68 | ???????????????????? stopService(intent); |
69 | ???????????????????? break ; |
70 | ???????????????? case R.id.Button03: |
72 | ???????????????????? bindService(intent,conn,Context.BIND_AUTO_CREATE); |
73 | ???????????????????? break ; |
74 | ???????????????? case R.id.Button04: |
76 | ???????????????????? unbindService(conn); |
77 | ???????????????????? break ; |
83 | ???????? b1.setOnClickListener(ocl); |
84 | ???????? b2.setOnClickListener(ocl); |
85 | ???????? b3.setOnClickListener(ocl); |
86 | ???????? b4.setOnClickListener(ocl);???? |
91 | ???? public void onDestroy(){ |
92 | ???????? super .onDestroy(); |
93 | ???????? Toast.makeText(MainHelloService. this , "MainHelloService onDestroy" , Toast.LENGTH_SHORT).show(); |
94 | ???????? Log.i(tag, "MainHelloService onDestroy" ); |
好了,本讲就到这里。
?