基本的图形组件View
No. | 组件名称 | 描述 |
1 | TextView | 表示一个文本的显示组件 |
2 | Button | 表示一个普通的按钮 |
3 | EditText | 表示一个可编辑的文本框组件 |
4 | CheckBox | 表示复选框组件 |
5 | RadioGroup | 表示单选钮组件 |
6 | Spinner | 下拉列表框 |
7 | DatePicker | 日期选择组件 |
8 | TimePicker | 时间选择组件 |
9 | ScrollView | 滚动条 |
10 | ProgressBar | 进度处理条 |
11 | SeekBar | 拖动条组件 |
12 | RatingBar | 评分组件 |
13 | ImageView | 图片显示组件 |
14 | ImageButton | 图片按钮 |
15 | AutoCompleteTextView | 自动完成文本组件 |
16 | Dialog | 对话框组件 |
17 | Toast | 信息提示框组件 |
18 | Menu | 菜单显示组件 |
组件的属性设置
No. | 属性名称 | 方法名称 | 描述 |
1 | android:background | public void setBackgroundResource (int resid) | 设置组件背景 |
2 | android:clickable | public void setClickable (boolean clickable) | 是否可以产生单击事件 |
3 | android:contentDescription | public void setContentDescription (CharSequence contentDescription) | 定义视图的内容描述 |
4 | android:drawingCacheQuality | public void setDrawingCacheQuality (int quality) | 设置绘图时所需要的缓冲区大小 |
5 | android:focusable | public void setFocusable (boolean focusable) | 设置是否可以获得焦点 |
6 | android:focusableInTouchMode | public void setFocusableInTouchMode (boolean focusableInTouchMode) | 在触摸模式下配置是否可以获得焦点 |
7 | android:id | public void setId (int id) | 设置组件ID |
8 | android:longClickable | public void setLongClickable (boolean longClickable) | 设置长按事件是否可用 |
9 | android:minHeight |
| 定义视图的最小高度 |
10 | android:minWidth | 定义视图的最小宽度 |
11 | android:padding | public void setPadding (int left, int top, int right, int bottom) | 填充所有的边缘 |
12 | android:paddingBottom | public void setPadding (int left, int top, int right, int bottom) | 填充下边缘 |
13 | android:paddingLeft | public void setPadding (int left, int top, int right, int bottom) | 填充左边缘 |
14 | android:paddingRight | public void setPadding (int left, int top, int right, int bottom) | 填充右边缘 |
15 | android:paddingTop | public void setPadding (int left, int top, int right, int bottom) | 填充上边缘 |
16 | android:scaleX | public void setScaleX (float scaleX) | 设置X轴缩放 |
17 | android:scaleY | public void setScaleY (float scaleY) | 设置Y轴缩放 |
18 | android:scrollbarSize |
| 设置滚动条大小 |
19 | android:scrollbarStyle | public void setScrollBarStyle (int style) | 设置滚动条样式 |
20 | android:visibility | public void setVisibility (int visibility) | 设置是否显示组件 |
21 | android:layout_width |
| 定义组件显示的宽度 |
22 | android:layout_height |
| 定义组件显示的长度 |
23 | android:layout_gravity |
| 组件文字的对齐位置 |
24 | android:layout_margin |
| 设置文字的边距 |
25 | android:layout_marginTop |
| 上边距 |
26 | android:layout_marginBottom |
| 下边距 |
27 | android:layout_marginLeft |
| 左边距 |
28 | android:layout_marginRight |
| 右边距 |
29 | android:background |
| 设置背景颜色 |
今天我么来看一下TextView 和EditText组件的基本使用。
TextView组件常用的设置方法
No. | 配置属性名称 | 对应方法 | 描述 |
1 | android:text | public final void setText (CharSequence text) | 定义组件的显示文字 |
2 | android:maxLength | public void setFilters (InputFilter[] filters) | 设置组件最大允许长度 |
3 | android:textColor | public void setTextColor (ColorStateList colors) | 设置组件的文本颜色 |
4 | android:textSize | public void setTextSize (float size) | 设置显示的文字大小 |
5 | android:textStyle |
| 设置文字显示的样式,粗体、斜体等 |
6 | android:selectAllOnFocus | public void setSelectAllOnFocus (boolean selectAllOnFocus) | 默认选中并获得焦点 |
7 | android:password | public final void setTransformationMethod (TransformationMethod method) | 按密文方式显示文本信息 |
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"//绝对布局 xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".MainActivity" > ? <TextView android:textColor="#ff00ffff//设置字体颜色 android:layout_height="wrap_content"//包裹内容 android:layout_width="wrap_content"//包裹内容 android:text="www.csdn.net"//显示内容 android:autoLink="all"//允许连接形式存在 android:background="#FF00FF00"//设置背景颜色 /></RelativeLayout>
在Android中所有的组件可以设置大小,但是在设置大小的时候需要指定其单位,这些单位如下:
px(pixels):像素;
dip(device independent pixels):依赖于设备的像素;
sp(scaled pixels—— best for text size):带比例的像素;
pt(points):点;
in(inches):英尺;
mm(millimeters):毫米。
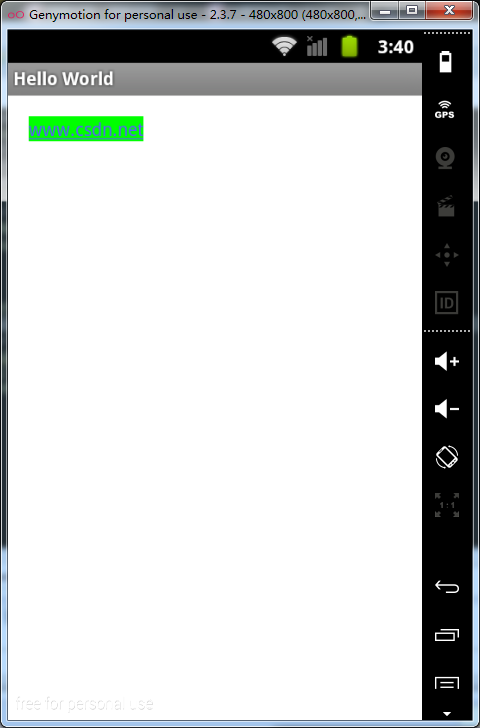
在JAVA文件中进行改变
package com.example.helloworld;import android.os.Bundle;import android.app.Activity;import android.widget.TextView;public class MainActivity extends Activity {private TextView info=null;//声明TextView @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); this.info=(TextView)super.findViewById(R.id.info);//找到textview info.setText("我在JAVA文件中已经改变了,tettview原来的值");//设置属性 info.setTextSize(20); } }
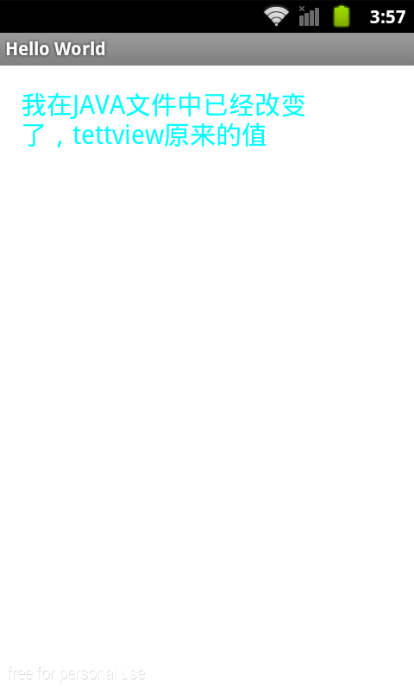
好了,txteview组件先简单介绍到这里,随后我们用到时候会具体介绍。
下节预报:Button组件的使用
- 1楼WTBEE4小时前
- 不错哦 整理的比较详细~~